

Programming Guides › Programming Guide for the Federation .NET SDK › Using the .NET SDK
Using the .NET SDK
This section contains the following topics:
Program Flow at the Asserting Party
Program Flow at the Relying Party
Federation Manager .NET SDK Logging
Programming Examples
.NET SDK Sample Application
Program Flow at the Asserting Party
With Federation Manager at the asserting party, a .NET application can provide Federation Manager with user identity information. Program flow with Federation Manager at the asserting party proceeds as follows:
- The .NET application calls the .NET SDK to generate an open format cookie with identity information.
- The .NET SDK returns an encrypted cookie. The key used to encrypt the cookie is derived from a shared secret, communicated between Federation Manager and the application out-of band.
- The .NET application sends the cookie to Federation Manager at the asserting party.
- Federation Manager receives and decrypts the cookie.
- Federation Manager extracts user identity information from the cookie.
- Optionally, Federation Manager can modify the cookie by updating or adding attributes.
- Federation Manager inserts the user identity information into a SAML Assertion.
The following diagram shows program flow at the asserting party:
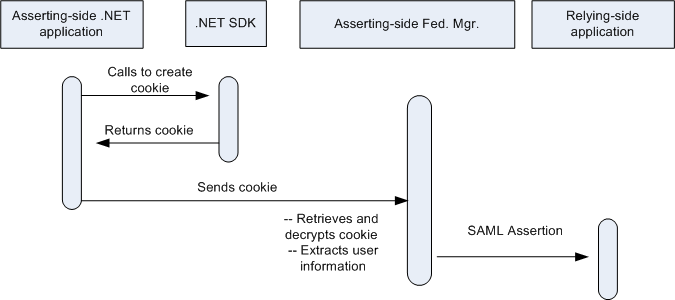
Program Flow at the Relying Party
With Federation Manager at the relying party, the .NET application can receive user information from Federation Manager. Program flow with Federation Manager at the relying party proceeds as follows:
- Federation Manager receives a SAML Assertion during request processing.
- Federation Manager creates the cookie with the latest user information.
- Federation Manager encrypts the cookie using a FIPS-compliant algorithm. The key used to encrypt the cookie is derived from a shared secret, communicated between Federation Manager and the application out-of band.
- Federation Manager sends the encrypted open format cookie to the .NET application.
- The .NET application calls the .NET SDK to decrypt and process the cookie.
- The .NET application retrieves values for assertion attributes and principal attributes.
- The .NET application can determine whether the cookie is no longer valid by calling the isExpired() method, with or without specifying a skew time. The method compares the expiration time stamp on the cookie, adding in the optional skew time, with the current GMT time. If the GMT time is greater, the cookie has expired. The cookie's expiration time stamp is specified using setTimeToLive() method when the cookie is created.
- The .NET application can also set URIs for AuthnContext and UserConsent.
The following diagram shows program flow at the relying party:
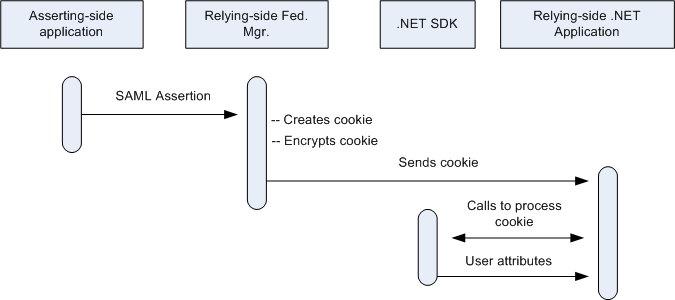
Federation Manager .NET SDK Logging
When enabled, .NET SDK logger writes messages to the standard output stream. Logging is disabled by default.
To enable Federation Manager .NET SDK logging
- Copy the Logger.xml file from the .NET SDK Installation directory\config and place it with the .NET SDK DLL the \bin folder.
- Set the EnableLogging parameter to yes in Logger.xml.
Logging is enabled.
Programming Examples
The following code fragments illustrate creating an open format cookie:
// Gets an object reference of the interface type IFederationOpenIdentity, bound to a custom
// implementation of the IFederationOpenIdentity interface.
// AES128/CBC/PKCS5Padding is the only supported cryptographic transformation string.
IFederationOpenIdentity openID = IdentityFactory.GetInstance("AES128/CBC/PKCS5Padding", UseHMACFlag);
// Initializes the parameters required to create cookie.
openID.InitCookieInfo(Domain, CookieZone, CookieName, Password);
// Sets a user attribute.
openID.LoginID = txtLoginID.Text;
// Creates an open format cookie and sets it into the response object.
openID.CreateCookie(HttpResponse);
The following code fragments illustrate consuming an open format cookie:
// Gets an object reference of the interface type IFederationOpenIdentity, bound to a custom
// implementation of the IFederationOpenIdentity interface.
// AES128/CBC/PKCS5Padding is the only supported cryptographic transformation string.
IFederationOpenIdentity openID = IdentityFactory.GetInstance("AES128/CBC/PKCS5Padding", UseHMACFlag);
// Initializes parameters needed to extract cookie.
openID.InitCookieInfo(Domain, CookieZone, CookieName, Password);
// Extracts the cookie from the HttpRequest, decrypts it, and saves the attributes in a Hashtable.
openID.ExtractCookie(HttpRequest);
// Retrieves some attributes.
String id = openID.LoginID;
String nid = openID.NameID;
.NET SDK Sample Application
The .NET test application generates an open format cookie and consumes it using the.NET SDK. The test application can be deployed in a number of ways. One suggested approach is listed following.
Note: Make sure the IIS Web Server is set to allow ASP .NET content.
To deploy the .NET SDK test application
- Create a folder (in this example, TestApplication).
- Copy the following files from the dotNet_SDK_home\testapp to your TestApplication folder:
- OpenCookieConsumer.aspx.cs
- OpenCookieConsumer.aspx
- OpenCookieConsumetUseHMAC.aspx.cs
- OpenCookieConsumetUseHMAC.aspx
- OpenCookieGenerator.aspx.cs
- OpenCookieGenerator.aspx
- web.config
- Create a bin folder in the TestApplication directory.
- Copy CA.Federation.FedIdentitySdk.dll from dotNet_SDK_home\bin to your TestApplication\bin.
- Open the web.config file to edit. In the <appSettings> section, change the Password, Zone, and Name keys.
- Password is the shared secret used to derive a cryptographic key
- Zone is the cookie zone.
- Name is the cookie name. The final name of the cookie generated includes the Zone and the Name.
- Go to the Internet Information Services Manager.
- Right click websites.
- Enter a description for the Web site.
- Assign a TCP port to the Web site (for example, 100).
- Enter or browse the path to the Web site home directory, that is, the location of the Test Application directory.
- On the Website Access Permissions dialog, select the Read and Run scripts (such as ASP) options.
- Select Finish.
- Restart IIS.
- Access the .NET SDK Test Application open format cookie creation page.
- Enter the login ID.
- Click Go.
The system displays the .NET SDK Test Application Open Format Cookie consumption page. The OpenCookieConsumer.aspx page displays the contents of the cookie. In this case, the only attribute in the cookie is Login ID.
- Access the .NET SDK Test Application Open Format Cookie consumption page, which decrypts the open format cookie and display the principal and assertion attributes contained in the cookie.
Copyright © 2012 CA Technologies.
All rights reserved.
 
|
|